Playwright Introduction
-
Eric Stanley
- January 11, 2023
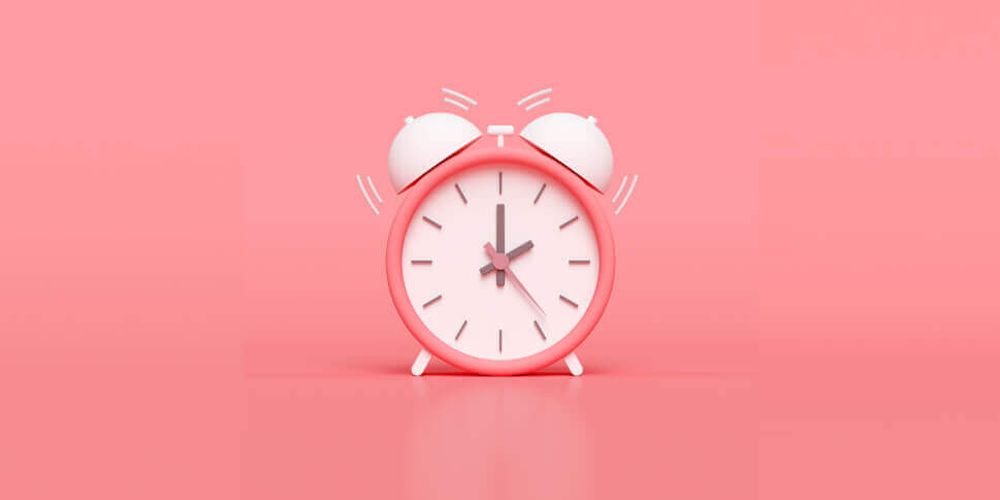
Automated testing has become an essential part of software development. It allows developers to ensure that their software meets the required quality standards and functionality, without the need for manual testing. There are many automated testing frameworks available today, each with its own set of features and benefits. In this blog post, we will focus on one such framework - Playwright
What is Playwright?
Playwright is an open-source testing framework developed by Microsoft. It is designed to automate end-to-end testing for web applications, including modern web frameworks such as React, Angular, and Vue. Playwright supports multiple programming languages, including JavaScript, TypeScript, Python, and C#
Why Use Playwright for Automated Testing?
There are several reasons why Playwright is an excellent choice for automated testing
- Cross-Browser Testing: Playwright supports automated testing across multiple browsers, including Chrome, Firefox, Safari, and Edge. It also allows developers to test web applications on different versions of the same browser
- Reliable Testing: Playwright is designed to be reliable. It has built-in mechanisms for handling flaky tests, such as automatic retries and waiting for elements to become visible
- Easy to Use: Playwright’s API is straightforward and easy to use. It allows developers to write tests in a clear and concise manner, reducing the learning curve
- Speed: Playwright is designed to be fast. It uses advanced techniques such as parallelization and page isolation to speed up test execution
Getting Started with Playwright
To get started with Playwright, you will need to install it using npm. You can install Playwright by running the following command
npm install playwright
Once you have installed Playwright, you can create a new test file and import the required modules
const { chromium } = require('playwright');
Next, you can create a new test case and launch the browser
(async () => {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
await page.goto('https://example.com');
await browser.close();
})();
The above code launches a new instance of the Chromium browser, creates a new browser context, and navigates to the example.com website. Finally, it closes the browser
Writing Tests with Playwright
Now that you have a basic understanding of how Playwright works, you can start writing tests. Playwright provides a simple and intuitive API for interacting with web pages. Here’s an example of how you can use Playwright to fill out a form and submit it
const { chromium } = require('playwright');
(async () => {
const browser = await chromium.launch();
const context = await browser.newContext();
const page = await context.newPage();
// Navigate to the form page
await page.goto('https://example.com/form');
// Fill out the form
await page.fill('input[name="name"]', 'John Doe');
await page.fill('input[name="email"]', 'john@example.com');
await page.fill('textarea[name="message"]', 'Hello, World!');
// Submit the form
await page.click('button[type="submit"]');
// Wait for the confirmation message
await page.waitForSelector('.confirmation-message');
// Verify the confirmation message
const message = await page.textContent('.confirmation-message');
expect(message).toContain('Thank you for submitting the form!');
// Close the browser
await browser.close();
})();
The above code navigates to a form page, fills out the form fields, submits the form, and then verifies the confirmation message
Conclusion
Automated testing is crucial for ensuring the quality and functionality of web applications. Playwright is a powerful and versatile testing framework that simplifies the process of writing automated tests. Its cross-browser support, reliability, and ease of use make it an excellent choice for both beginners and experienced developers
By leveraging Playwright’s features, developers can save time and effort by automating repetitive tasks, catching bugs early in the development cycle, and delivering high-quality software to end-users. So, if you haven’t already, give Playwright a try and experience the benefits of automated testing in your web development projects