Simplifying Element Selection in JavaScript with CSS for Automation Testing using Playwright
-
Eric Stanley
- February 15, 2023
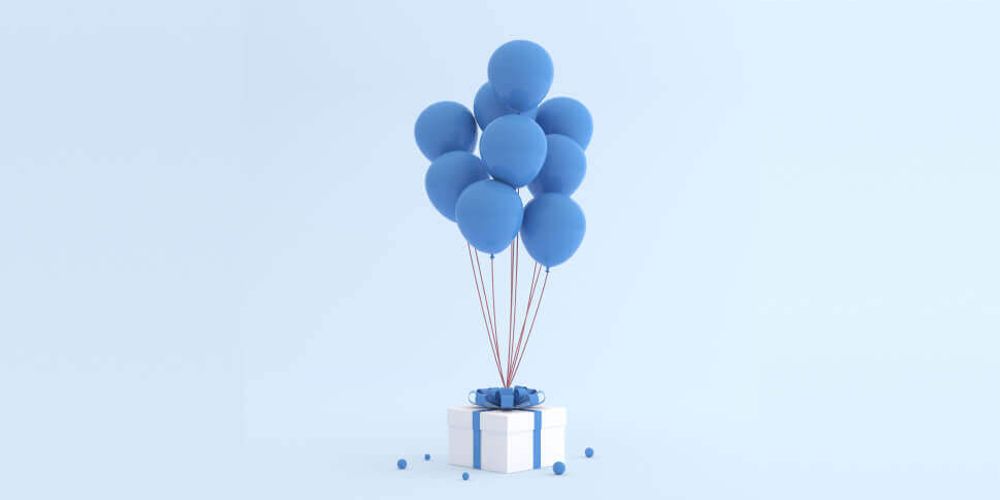
Introduction
When it comes to automation testing using Playwright, the ability to efficiently select elements on a web page becomes crucial. CSS selectors offer a powerful and flexible way to identify and interact with elements. In this blog post, we will explore various ways to select elements using CSS in JavaScript, with a focus on automation testing using Playwright. Along the way, we will provide practical examples to illustrate each method. Let’s dive in!
Selecting elements by ID
The simplest and most efficient way to select an element is by its ID. In CSS, IDs are unique identifiers assigned to elements. By leveraging the page.$()
method in Playwright, we can select an element using its ID as follows
const element = await page.$('#elementId');
Selecting elements by class
CSS classes provide a convenient way to group elements. To select elements by class, we can use the page.$$()
method in Playwright, which returns an array of elements matching the provided class name
const elements = await page.$$('.className');
Selecting elements by tag name
To select elements based on their HTML tag name, we can use the page.$$('tagName')
method. This approach is especially useful when we want to interact with multiple elements of the same type on a page
const elements = await page.$$('input');
Selecting elements by attribute
CSS selectors allow us to target elements based on their attributes. We can use attribute selectors such as [attribute=value]
or [attribute*=value]
to match elements with specific attribute values or containing a specific value
const element = await page.$('[name="email"]');
const elements = await page.$$('a[href*="example.com"]');
Selecting nested elements
To select elements that are nested within other elements, we can use the combination of CSS selectors. By specifying the parent element followed by a space and the child element, we can target the desired nested elements
const nestedElement = await page.$('.parentClass .childClass');
Selecting elements using advanced CSS selectors
CSS provides a wide range of advanced selectors that allow us to target elements based on specific conditions. Some examples include :nth-child
, :first-of-type
, :last-of-type,
:not,
and many more. These advanced selectors can be powerful tools in our automation testing arsenal
const oddElements = await page.$$('ul li:nth-child(odd)');
const lastElement = await page.$('div:not(.ignore)');
Conclusion
Efficiently selecting elements is crucial for successful automation testing using Playwright. CSS selectors provide a versatile and powerful way to identify elements on a web page. In this blog post, we have explored various techniques to select elements using CSS in JavaScript, with a focus on automation testing. By utilizing these methods and combining them with Playwright’s robust functionality, you can streamline your automation testing efforts and improve the reliability of your tests. Happy testing!
Remember, automation testing requires not only selecting elements but also interacting with them. Playwright offers a rich set of APIs to perform actions on the selected elements, making it an excellent choice for automation testing